Saw a question raised up by a member in Fluent Form official Facebook community group. Objective is to ensure the zip code entered by visitor is numeric instead of alphanumeric. Let me share this by using Fluent Form hooks and some css + jQuery.
Fluent Form – The WordPress Best Form Builder
I could say, Fluent Form is the best WordPress form builder. It comes with free and pro version (no affiliate). I have no regret I invested on this Pro plugin in my life. Their codes are clean and well documented, highly recommended for WordPress web agency or WordPress developer. I am able to make use of this superb form builder plugin to achieve many different scenarios requested by my customers without spending more on other plugins.
Alright, just wanted to share how happy am I to this plugin. Without wasting time, let’s get our hand dirty now.
Step 1 – Change Input Type
If you inspect the zip code field in developer tool, you will noticed that it’s a text input type. I am guessing the reason why Fluent Form team not change it as number type input is that could be some country’s zip code contains alphabet.
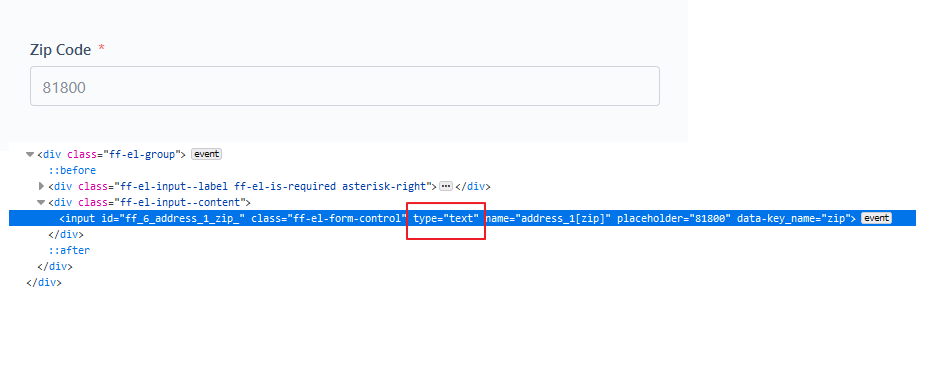
Thanks to Fluent Form team, we can change this by using the fluentform_rendering_field_data_elementName
filter hook.
<?php
// Change the address field rendering data for address
add_filter( 'fluentform_rendering_field_data_address', function( $data, $form ){
// I just want to target my form ID 6
$targetFormId = 6;
if( $targetFormId != $form->id ) {
return $data;
}
// at this point, you can var_dump() or use dd() function by Fluent Form plugin to show the $data array for troubleshooting purposes
// dd($data);
// easily change the type attribute to number
$data['fields']['zip']['attributes']['type'] = 'number';
// inputmode is to show number pad in mobile device
$data['fields']['zip']['attributes']['inputmode'] = 'numeric';
return $data;
}, 10, 2);
?>
After applying above filter, you will be able to see the zip code field now rendered as number type input.
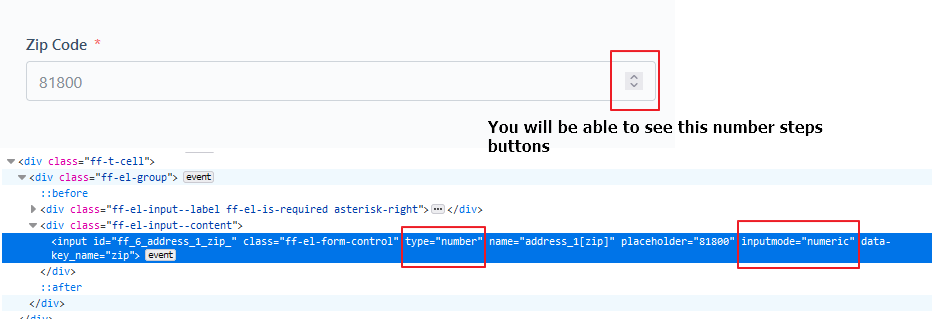
Step 2 – Apply CSS
Well, the default number type input field will be showing number steps button on different browser. Let’s remove this by tweaking on the css part. You can apply below css in the Fluent Form custom css section.
/*
* Assuming your address element attribute name is address_1
* .fluent_form_FF_ID only can be using in Fluent Form Custom CSS section
*/
.fluent_form_FF_ID input[name="address_1[zip]"] {
-webkit-appearance: textfield;
-moz-appearance: textfield;
appearance: textfield;
}
/* This is to disable the Chrome number step button */
.fluent_form_FF_ID input[name="address_1[zip]"]::-webkit-outer-spin-button,
.fluent_form_FF_ID input[name="address_1[zip]"]::-webkit-inner-spin-button {
-webkit-appearance: none;
}
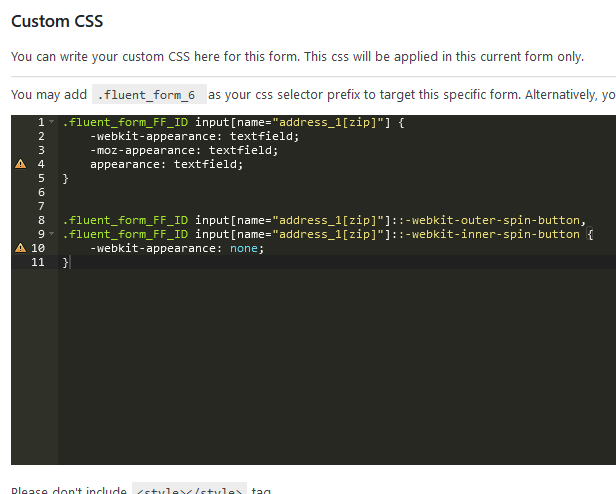
Please note that this css also can be applied on a normal number type field not only limited to Fluent Form. Just change the selector to your desired one will do.
Step 3 – Apply Jquery (Optional)
Next problem, if you are using browser like Firefox, actually you can input non-numeric value in this field before submit. Of course, it will be stopped by the browser when you hit submit button.
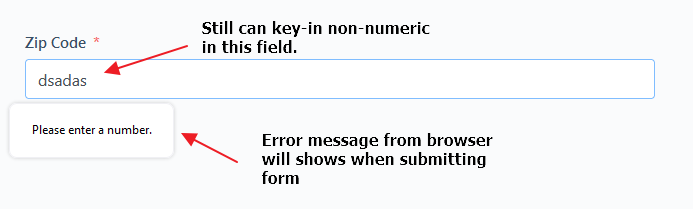
If this is bothering you, the only way is to apply below jquery on the correct selector.
jQuery(document).on('keypress', 'input[name="address_1[zip]"]', function(evt){
return evt.charCode >= 48 && evt.charCode <= 57;
})
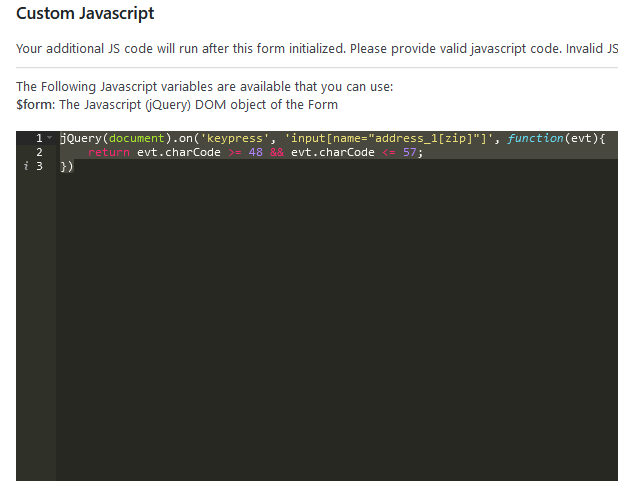
Now, you could see the zip code field working nice. Actually you might be able to stop at this stage as browser should stops them to submit the form if the input value is not numeric.
However, this is not the recommended way because the validation only working on frontend. Which means, someone might be able to submit the zip code in alphanumeric value. Don’t ask me who are those “someone”, but it’s possible!
Step 4 – Backend Validation (Recommended)
As per my perfectionism characteristic, I will prefer to validate the zip code in backend just in case the frontend form meet some unexpected scenarios or errors.
We will be using fluentform_validate_input_item_input_key
and fluentform_validation_errors
filter hooks to achieve this.
<?php
// Additional validation for address field
add_filter( 'fluentform_validate_input_item_address', function( $errorMessage, $field, $formData, $fields, $form, $errors ) {
// I just want to target my form ID 6
$targetFormId = 6;
if( $targetFormId != $form->id ) {
return $errorMessage;
}
$currentFieldName = $field['name'];
// Only target my field with attribute name is address_1
if( $currentFieldName != 'address_1' ) {
return $errorMessage;
}
$zip = $formData['address_1']['zip'];
if ( !is_numeric($zip) ) {
return array('zip'=>"Must be numeric zip code.");
}
return $errorMessage;
}, 10, 6);
?>
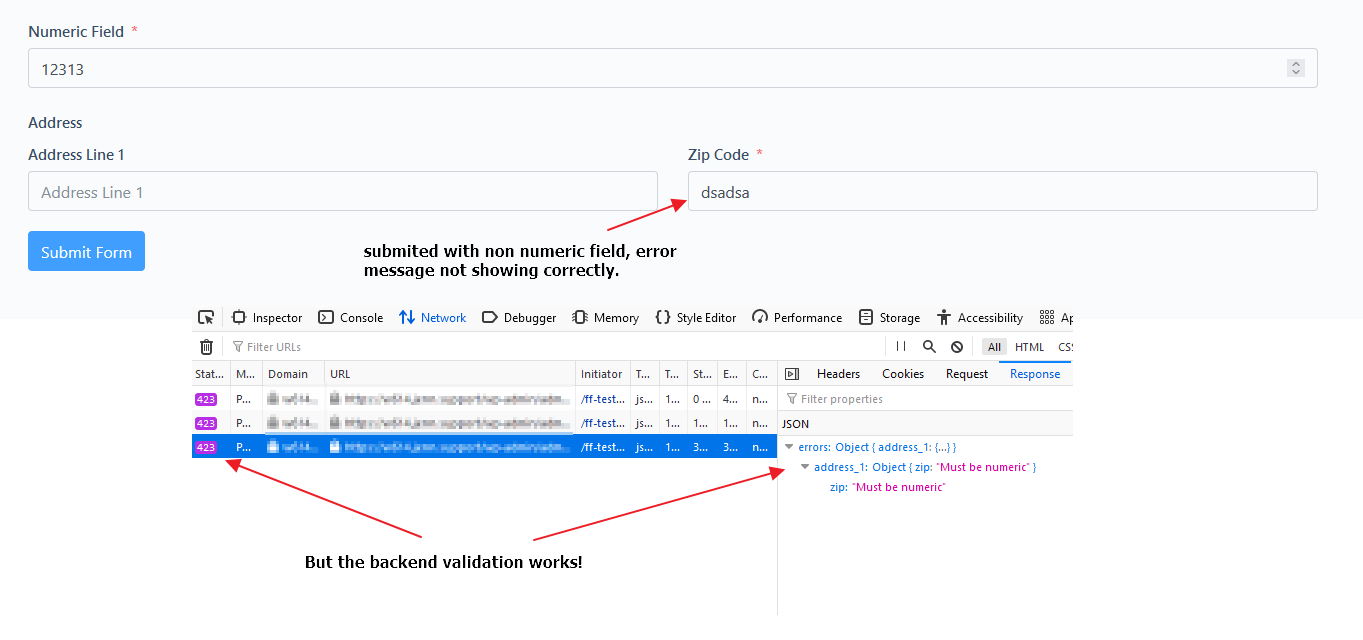
Now, if someone managed to submit the zip code in non-numeric value, Fluent Form will reject with errors. But the errors will not render on the zip code field for now. Let’s fix it.
<?php
// Fix the error message if errors detected
add_filter( 'fluentform_validation_errors', function( $errors, $formData, $form, $fields ){
// I just want to target my form ID 6
$targetFormId = 6;
if ( $targetFormId != $form->id ) {
return $errors;
}
// Loop through the errors and assign to the correct key so that the error message can display on zip field
if( isset( $errors['address_1'] ) && is_array( $errors['address_1'] )) {
foreach( $errors['address_1'] as $key=>$value ) {
$name = "address_1[{$key}]";
$errors[$name] = $value;
}
}
return $errors;
}, 10, 4);
?>
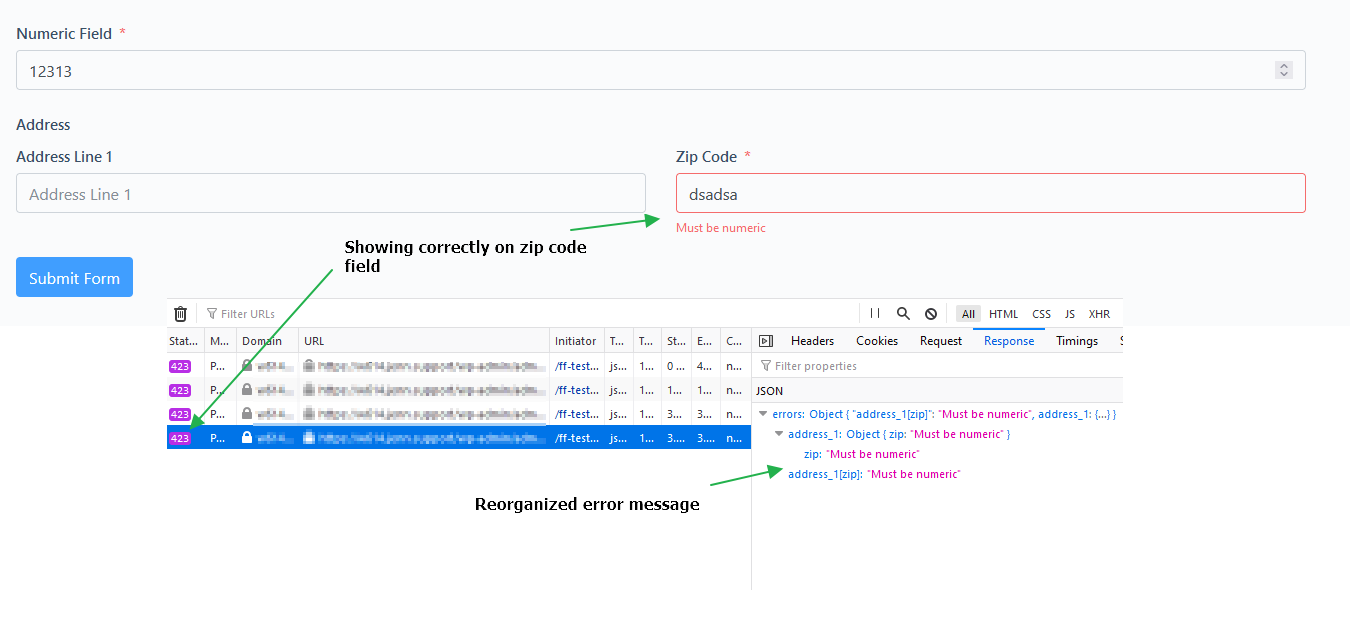
Hurray! Now my address zip code field is validated perfectly!
Conclusion
Fluent Form team (WPManageNinja) using a lots of filters and actions in the plugin, this bring a lots of beneficial to the users like us! You are welcome to contact me if you want more examples or tutorials like this.
Fluent Form Filters / Actions used:
fluentform_validate_input_item_address
fluentform_validation_errors
fluentform_rendering_field_data_address
Reference: Fluent Form Developer Docs