Instead of manually clicking the purge cache button in WordPress admin panel, I wish to purge the cache by using code or apply on my own logic. Let me share my way in this article.
WordPress LiteSpeed Cache (LSCWP) is a very powerful cache plugin. Majority WordPress website hosted on Litespeed server will be using it. If you are using WooCommerce, cache playing an important role but you really need to be careful when using it as well. Page cache could be your friend and could be your most headache enemy if you not do it right.
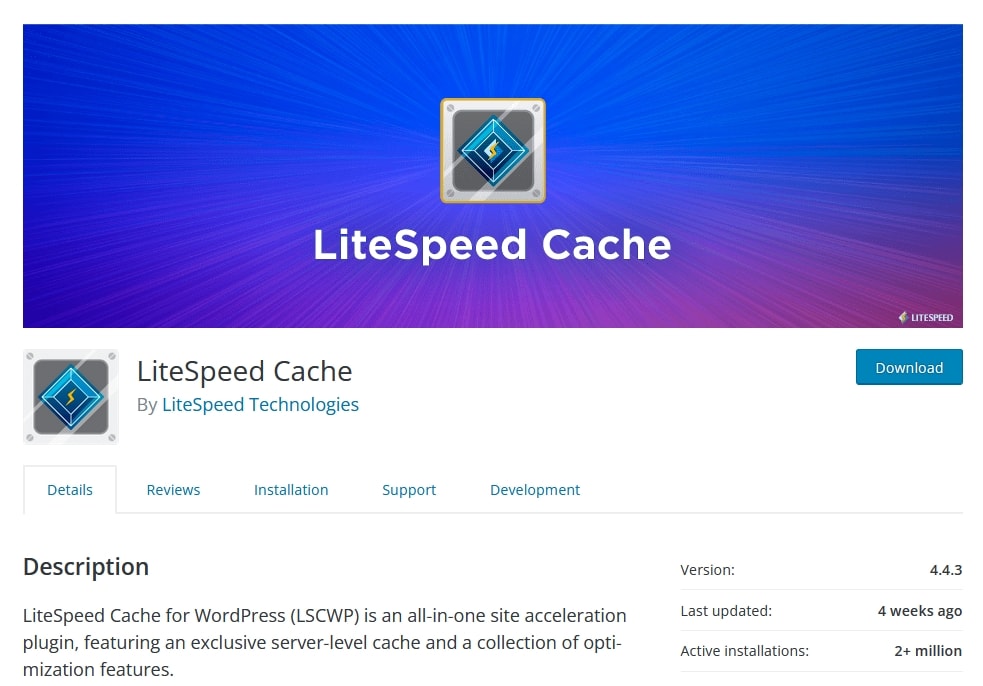
Use Case / Problem
I believe your WooCommerce website will organize promotion for a particular time period, like Black Friday sales from date A to date B. WooCommerce owner then will use some dynamic discount plugins to set the promotion with date A to date B easily by clicking a few buttons.
However, if you are using page cache plugin, you might encounter problem like the promotion or discount price did not show up when date A reached. This might be causing due to the particular page cache was generated before date A and the page cache not expired yet (being served), and that’s why your visitor will still see the product page with original price.
Yayaya, I knew when visitors add those discounted products to cart, they still will be getting the discounted price (cart and checkout page usually configured as cache excluded pages), but is this what you want?
Hence, programmatically purge cache is needed. If it’s configured as scheduled will be great as well! Like scheduling a daily purge all action exactly at 00:00:00 in your website timezone. Then the above issue will be solved.
Trigger The Purge
By using below code, you could purge all caches in LiteSpeed Cache for WordPress. You could try to use it on a custom shortcode for testing purpose.
<?php
// wrap this in a function
if (class_exists('\LiteSpeed\Purge')) {
\LiteSpeed\Purge::purge_all();
}
// another way
if (class_exists('\LiteSpeed\Purge')) {
do_action('litespeed_purge_all');
}
?>
Set Schedule In WP Cron
Next, let’s create a schedule in WP Cron. Please note that you must also configure to use server cronjob to execute WP Cron so that the scheduled WP Cron will be executed on time. Otherwise, this will be a failed because default WP Cron only triggered when a visitor land on your website.
<?php
// Register a new cron schedule with 86400 seconds interval
add_filter( 'cron_schedules', 'add_itchycode_cron_schedule' );
function add_itchycode_cron_schedule( $schedules ) {
if(!isset($schedules["itchycode_daily"])){
$schedules['itchycode_daily'] = array(
'interval' => 86400,
'display' => esc_html__( 'Itchycode Every Day' ), );
}
return $schedules;
}
// Schedule our custom job, I am going to set it to execute everyday at 00:00:05 (GMT+8)
// This timing is to ensure the 1st visitor visit my site will be after a new day based on my promotion behaviour (start date + end date)
if ( ! wp_next_scheduled( 'itchycode_daily' ) ) {
wp_schedule_event( strtotime('00:00:05 Asia/Kuala_Lumpur'), 'itchycode_daily', 'itchycode_daily' );
}
// Now let's hook it up with our function
add_action( 'itchycode_daily','clear_litespeed_cache' );
function clear_litespeed_cache() {
if (class_exists('\LiteSpeed\Purge')) {
\LiteSpeed\Purge::purge_all();
}
}
?>
Alright, actually the code will work as expected, which will be purging all caches in LiteSpeed Cache for WordPress. But how are you going to check it?
WP Control To Check All Scheduled Tasks
WP Control plugin is my “must have” plugin in every WordPress website. I will use it to check whether my WP Cronjobs executed properly or not, check any weird tasks scheduled by other plugins etc.
Now, just head to Tools > Cron Events, your custom scheduled task will be showing there.
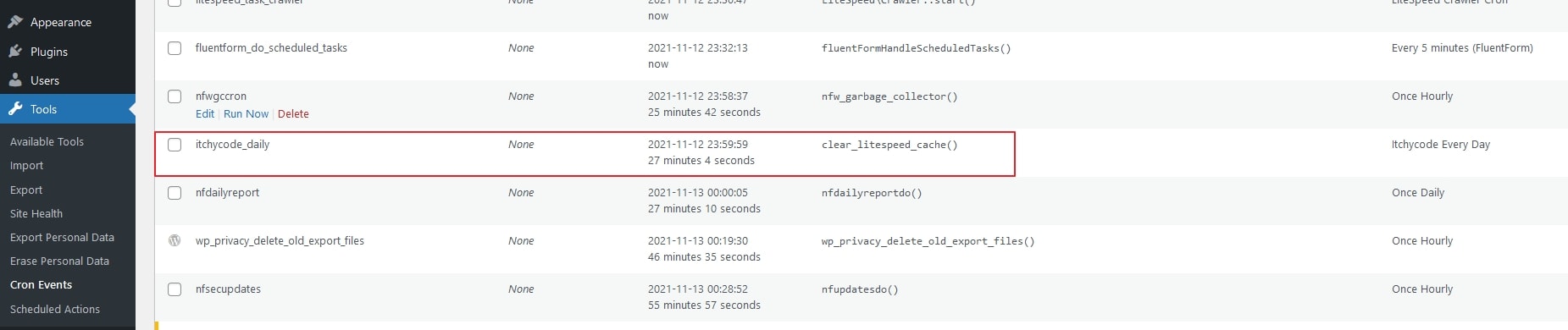
Summary
If you are not using LiteSpeed Cache for WordPress, you will still be able to do the same as long as you found the way to purge the cache programmatically. Once got it, just use the same way to schedule the task, and all should be working smooth. I will share the way to purge Swift Performance cache next time too.
Updated 2022 Feb: Purge Swift Performance in coding way published.
WordPress functions / hooks being used this example:
cron_schedules
wp_next_scheduled
litespeed_purge_all
\LiteSpeed\Purge::purge_all()