First share in 2022. As promised, let me share the coding way to clear Swift Performance cache in WordPress.
Swift Performance is my another favorite caching plugin in WordPress. Usually I will use it if the WordPress site hosted on non Litespeed server, it works beautiful and perfectly on Nginx. You can try the free Swift Performance Lite from WordPress repository.
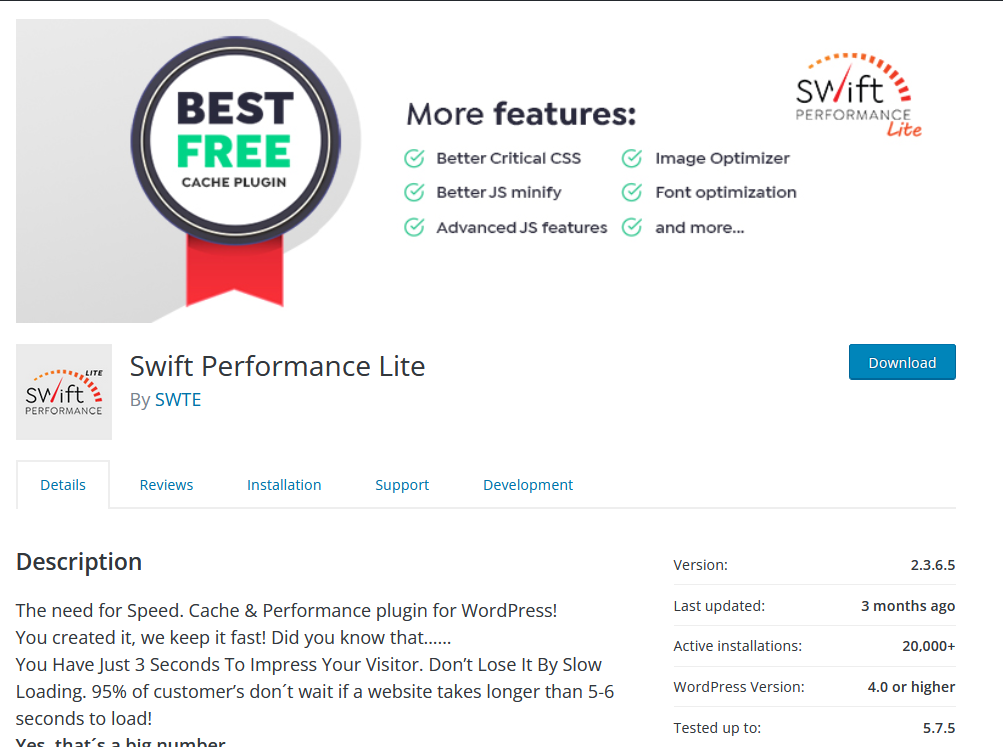
Trigger The Purge
If you dig into the Swift Performance code, you could use the clear_all_cache()
function as below.
<?php
if (class_exists('Swift_Performance_Cache')) {
Swift_Performance_Cache::clear_all_cache();
}
?>
If you wrap the above code into a shortcode and execute it, you should be able to see the Swift Performance warmup table showing missing cache for all links, which means you successfully triggered the purge!
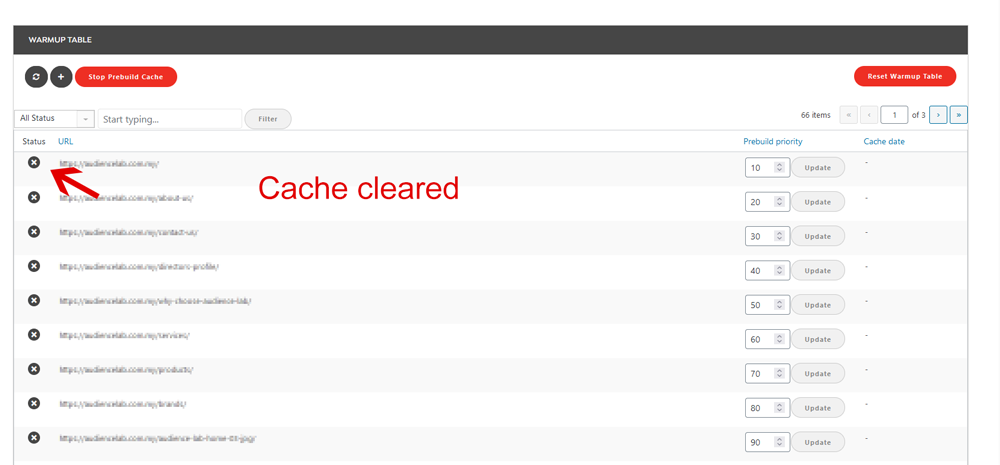
Remove and Rebuild Warmup Table
It might be a case that you need to rebuild the warmup table in Swift Performance. Just have a look to the codes below.
<?php
// Remove the Swift Performance Warmup Table
global $wpdb;
$wpdb->query('DROP TABLE IF EXISTS ' . SWIFT_PERFORMANCE_TABLE_PREFIX . 'warmup');
delete_option(SWIFT_PERFORMANCE_TABLE_PREFIX . 'db_version');
// Remove the transient so we can prebuild the url again
delete_transient('swift_performance_initial_prebuild_links');
?>
Now, let’s rebuild the warmup table.
<?php
if (class_exists('Swift_Performance_Cache')) {
Swift_Performance::db_install();
Swift_Performance::get_prebuild_urls();
}
?>
Bundle Up As Single Function
After understand the concepts and simple functions above, let’s bundle it up. And I will execute this function to my scheduled WP Cron as per my last tutorial.
<?php
// Hook on my daily scheduled WP Cronjob, which will be running on 00:00:05 daily
add_action( 'itchycode_daily','clear_swift_performance_cache' );
function clear_swift_performance_cache() {
if (class_exists('Swift_Performance_Cache')) {
global $wpdb;
$wpdb->query('DROP TABLE IF EXISTS ' . SWIFT_PERFORMANCE_TABLE_PREFIX . 'warmup');
delete_option(SWIFT_PERFORMANCE_TABLE_PREFIX . 'db_version');
delete_transient('swift_performance_initial_prebuild_links');
Swift_Performance::db_install();
Swift_Performance_Cache::clear_all_cache();
Swift_Performance::get_prebuild_urls();
}
}
?>
Summary
Swift Performance and LiteSpeed Cache are my preferred caching plugin. It’s my pleasure if this article could give a help on your WordPress website.
WordPress functions / hooks being used this example:
Swift_Performance_Cache::clear_all_cache()
Swift_Performance::db_install()
Swift_Performance::get_prebuild_urls()