Imagine how cool it is if we can use Fluent Forms to suggest some WooCommerce products after visitor answered a few simple questions. Some more, it’s without additional plugin.
Fluent Forms
Yea, Fluent Forms plugin is my favorite WordPress form builder as always. WP Manage Ninja just released latest version (4.3.5) and added a very interesting module — Quiz module! (Only for Pro version) I will play around with this module later for sure. But now let us focus on the WooCommerce products suggestion feature first!
Environment Setup
- WordPress 5.9.3
- PHP 7.4
- Open LiteSpeed Server
- Hello Theme by Elementor (You can use any theme you like)
- Fluent Forms Free 4.3.5
- Fluent Forms Pro 4.3.5 (Optional)
- WooCommerce 6.4.1
- All custom codes in this tutorial place in theme / child theme functions.php
I created several WooCommerce products in my test website so I could use them as suggested products later on.
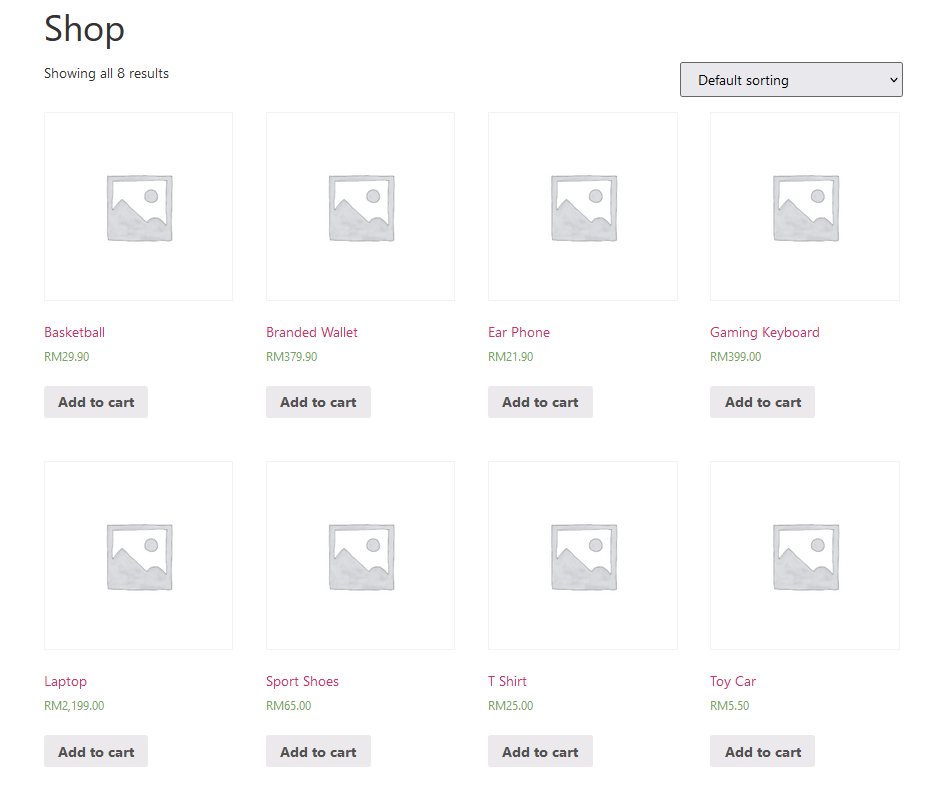
Step 1 – Plan Your Product Suggestion Logic
We must set a product suggestion logic first. So mine will be as below.
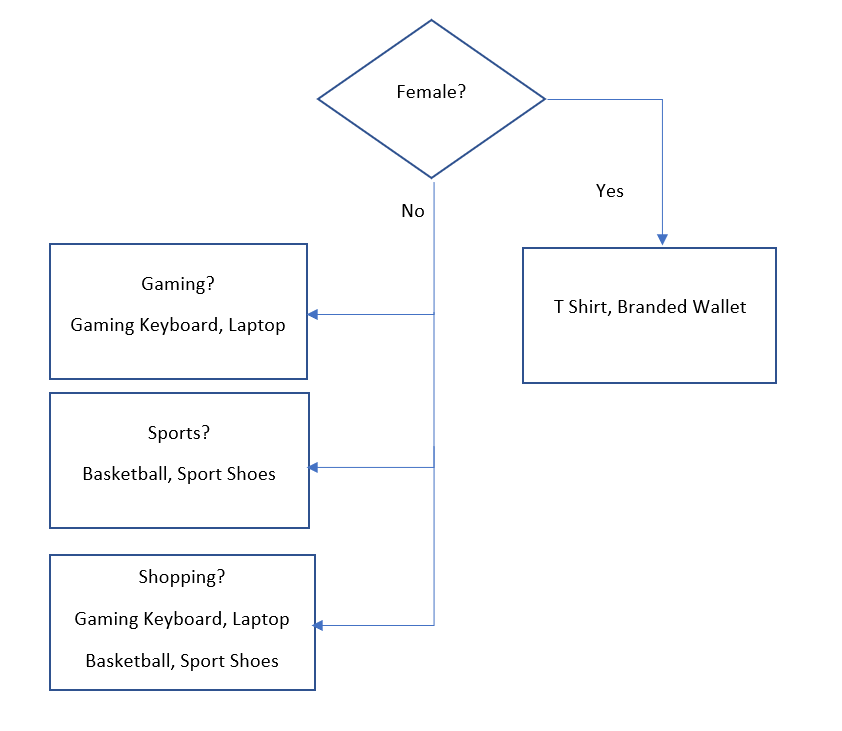
Logic explanation:
As long as the gender is female, my form will suggests T shirt and branded wallet. If visitor is male, suggests gaming keyboard and laptop if hobby is Gaming, suggests basketball and sport shoes if hobby is Sports, suggests all 4 products if hobby is Shopping.
Step 2 – Create Form
Once plan and logic set, I can easily create a product suggestion form with 2 questions. Ask for visitor’s hobby and gender. I set both as radio input, required, and given the name attribute accurately so we can code it out smooth later. Please avoid to name them as radio_input_1 or input_1 etc, this will be confusing yourself as the coder.
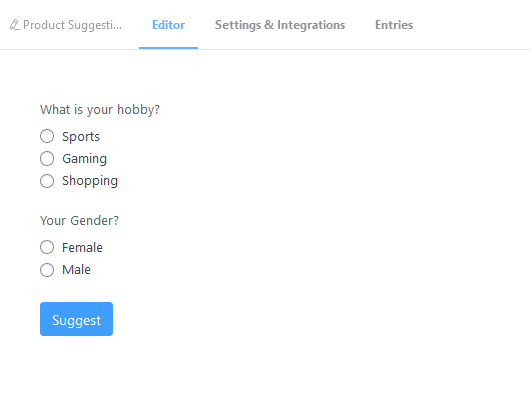
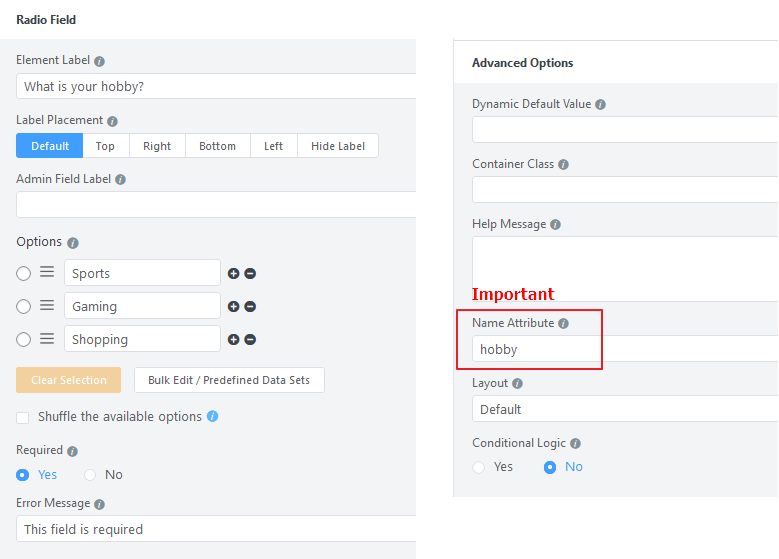
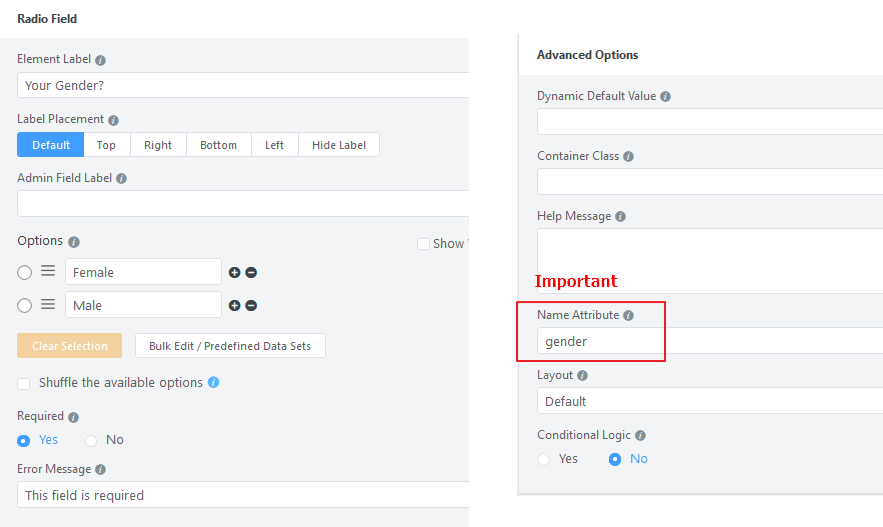
After saved the form, switch to the Settings & Integrations tab, I will activate the Deny empty submission option. If you are using Fluent Forms Pro version, you could activate the Delete entry data after form submission or Enable auto delete old entries too since this is a product suggestion form and the submission not necessary to keep. (Of course you can keep If you want to analyze your visitor submission.)
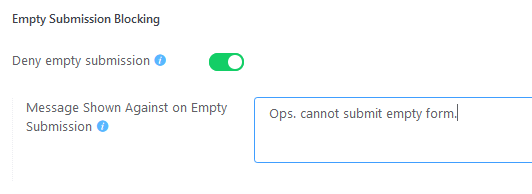
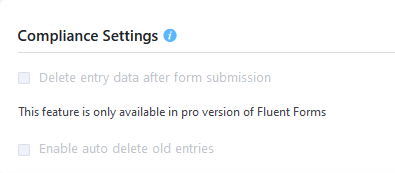
Now, you can use the shortcode [fluentform id="YOUR_FORM_ID"
] to embed it on any page you like. I just create a simple page and paste the shortcode into it.
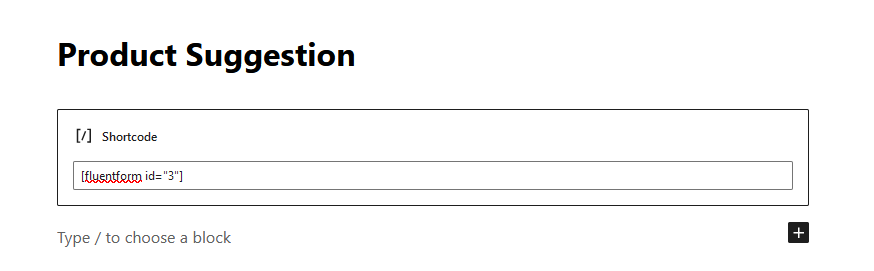
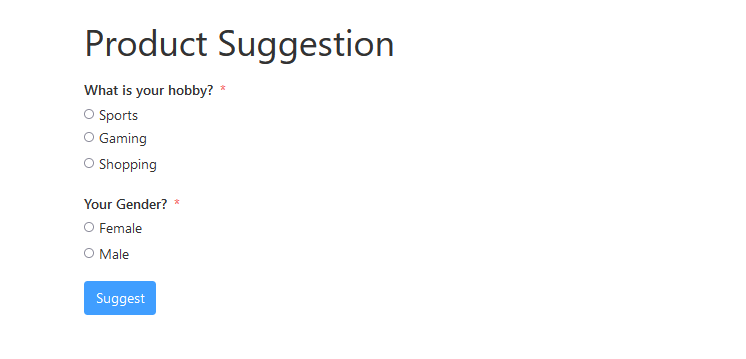
Step 3 – Use Fluent Forms Hooks
Okay, the required validations just let Fluent Form to handle. We just need to get the visitor answers from fluentform_before_insert_submission
action hook.
<?php
add_action( 'fluentform_before_insert_submission', 'itchycode_suggest_products', 10, 3 );
function itchycode_suggest_products( $insertData, $data, $form ) {
// My product suggestion form id is 3
$my_form_id = 3;
if( $form->id != $my_form_id ) {
return;
}
// Get the visitor answers from $data
$hobby = $data['hobby'];
$gender = $data['gender'];
// Suggested product ids will be saved in this array
$product_ids = array();
/**
* My WooCommerce product IDs as below:
* Sport Shoes => 99
* Basketball => 98
* Branded Wallet => 97
* Gaming Keyboard => 96
* Laptop => 95
* T Shirt => 69
*/
if( $gender == 'Female' ) {
// Female will suggest T Shirt and Branded Wallet only
$product_ids = [69, 97];
} else {
// Male
switch( $hobby ) {
case 'Sports':
// $hobby === 'Sports', suggest Basketball and Sport Shoes
$product_ids = [98, 99];
break;
case 'Gaming':
// $hobby === 'Gaming', suggest Gaming Keyboard and Laptop
$product_ids = [96, 95];
break;
case 'Shopping':
default:
// $hobby === 'Gaming', suggest Basketball, Sport Shoes, Gaming Keyboard and Laptop
$product_ids = [98, 99, 96, 95];
break;
}
}
}
?>
Please read thru the comments and you will understand it easily. However, the form still do nothing because we just populate our product IDs without next action. How to display those products after the form submission?
Step 4 – Use WooCommerce products shortcode
The easiest way to display WooCommerce products is using it’s prebuilt shortcode. You could read more in their documentation page.
// This will output products by ID
[products ids="98,99,96,95"]
We will also use fluentform_submission_confirmation
filter hook to replace the confirmation message with our WooCommerce products.
Final code as below. (Additional codes started on line 53.)
<?php
add_action( 'fluentform_before_insert_submission', 'itchycode_suggest_products', 10, 3 );
function itchycode_suggest_products( $insertData, $data, $form ) {
// My product suggestion form id is 3
$my_form_id = 3;
if( $form->id != $my_form_id ) {
return;
}
// Get the visitor answers from $data
$hobby = $data['hobby'];
$gender = $data['gender'];
// Suggested product ids will be saved in this array
$product_ids = array();
/**
* My WooCommerce product IDs as below:
* Sport Shoes => 99
* Basketball => 98
* Branded Wallet => 97
* Gaming Keyboard => 96
* Laptop => 95
* T Shirt => 69
*/
if( $gender == 'Female' ) {
// Female will suggest T Shirt and Branded Wallet only
$product_ids = [69, 97];
} else {
// Male
switch( $hobby ) {
case 'Sports':
// $hobby === 'Sports', suggest Basketball and Sport Shoes
$product_ids = [98, 99];
break;
case 'Gaming':
// $hobby === 'Gaming', suggest Gaming Keyboard and Laptop
$product_ids = [96, 95];
break;
case 'Shopping':
default:
// $hobby === 'Gaming', suggest Basketball, Sport Shoes, Gaming Keyboard and Laptop
$product_ids = [98, 99, 96, 95];
break;
}
}
add_filter( 'fluentform_submission_confirmation', function( $returnData, $form, $confirmation ) use ( $my_form_id, $product_ids) {
// Only target my product suggestion form
if( $form->id!= $my_form_id ) {
return $returnData;
}
// Convert our product ids into string with comma separator
$ids = implode(',', $product_ids);
$limit = count($product_ids);
$columns = 4;
// Execute the products shortcode by WooCommerce
ob_start();
echo do_shortcode("[products limit='{$limit}' columns='{$columns}' ids='{$ids}']");
$wc_products = ob_get_clean();
// Replace the confirmation message with our WooCommerce products
$returnData = [
'message' => $wc_products,
'action' => 'hide_form',
];
return $returnData;
}, 10, 3);
}
?>
Great! Let’s check our result in frontend now!
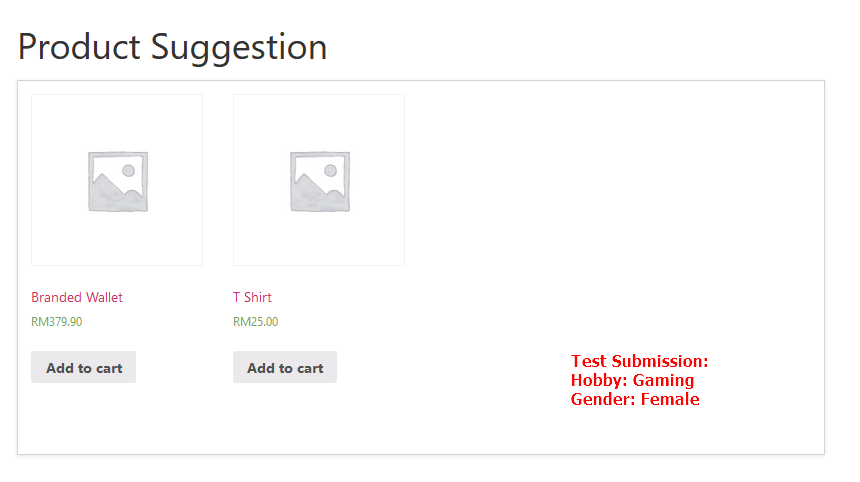
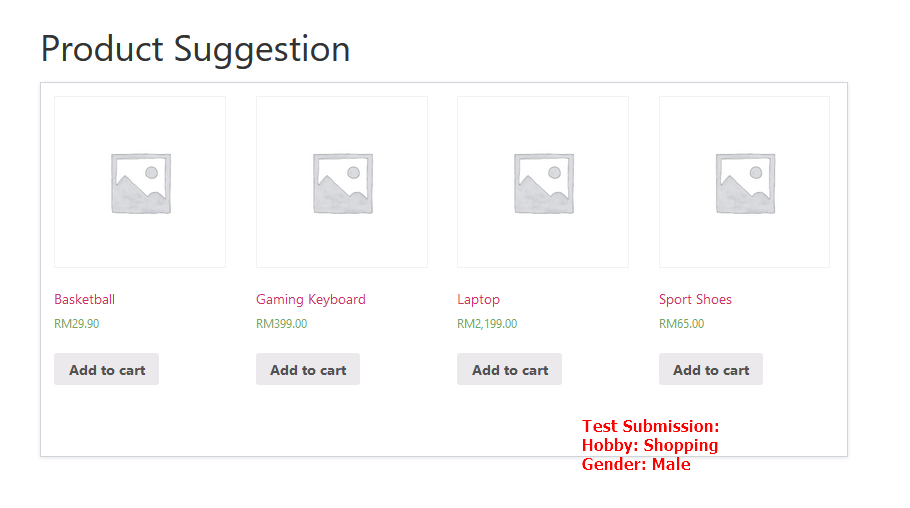
Work like a charm!!
Conclusion
This is just a simple customization to connect different plugins and work together. I am always suggest WordPress users to try use your existing installed plugins to solve your problem. Learn and understand your existing plugins is a must. Try not to install new plugin for every single new requirement. The more plugins you have, the more efforts needed to maintain your WordPress website.
Hope my article and sharing can help a little on your current problem. Welcome to connect with me and please please correct me if any code in this article is wrong or there is better approach.
Action & Filter hooks used in this tutorial:
fluentform_before_insert_submission
fluentform_submission_confirmation
- [
products
] shortcode